- 积分
- 2
贡献16
飞刀0 FD
注册时间2014-9-24
在线时间0 小时
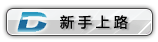
|
发表于 2014-9-24 18:23:54
|
显示全部楼层
随便找了个程序试了一下 好像接受到0x13不会挂起。
图上不了 就不传了- #include <stdio.h>
- #include <unistd.h>
- #include <stdlib.h>
- #include <string.h>
- #include <sys/types.h>
- #include <errno.h>
- #include <termios.h>
- #include <sys/stat.h>
- int set_opt(int fd, int nSpeed, int nBits, char nEvent, int nStop)
- {
- struct termios newtio, oldtio;
- /*保存测试现有串口参数设置,在这里如果串口号等出错,会有相关的出错信息*/
- if(tcgetattr(fd, &oldtio) != 0)
- {
- perror("SetupSerial 1");
- return -1;
- }
- bzero(&newtio, sizeof(newtio));
- /*一 设置字符大小*/
- newtio.c_cflag |= CLOCAL | CREAD;
- // newtio.c_cflag &= ~CSIZE;
- newtio.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
- newtio.c_oflag &= ~OPOST;
- newtio.c_iflag &= ~IXON;
- newtio.c_iflag |= IXANY;
- /*设置数据位 */
- switch(nBits)
- {
- case 7:
- newtio.c_cflag |= CS7;
- break;
- case 8:
- newtio.c_cflag |= CS8;
- break;
- }
- /*设置奇偶校验位*/
- switch(nEvent)
- {
- case 'O'://奇数
- newtio.c_cflag |= PARENB ;
- newtio.c_cflag |= PARODD ;
- newtio.c_iflag |= (INPCK | ISTRIP);
- break;
- case 'E'://偶数
- newtio.c_cflag |= PARENB ;
- newtio.c_cflag &= ~PARODD ;
- newtio.c_iflag |= (INPCK | ISTRIP);
- break;
- case 'N':// 无奇偶校验位
- newtio.c_cflag &= ~PARENB ;
- break;
- }
- /*设置波特率*/
- switch(nSpeed)
- {
- case 4800:
- cfsetispeed(&newtio, B4800);
- cfsetospeed(&newtio, B4800);
- break;
- case 9600:
- cfsetispeed(&newtio, B9600);
- cfsetospeed(&newtio, B9600);
- break;
- case 19200:
- cfsetispeed(&newtio, B19200);
- cfsetospeed(&newtio, B19200);
- break;
- case 57600:
- cfsetispeed(&newtio, B57600);
- cfsetospeed(&newtio, B57600);
- break;
- case 115200:
- cfsetispeed(&newtio, B115200);
- cfsetospeed(&newtio, B115200);
- break;
- default:
- cfsetispeed(&newtio, B115200);
- cfsetospeed(&newtio, B115200);
- break;
- }
- /*设置停止位 */
- if(1 == nStop)
- newtio.c_cflag &= ~CSTOPB;
- else if(2 == nStop)
- newtio.c_cflag |= CSTOPB;
- /*设置等待时间和最小接收字符 */
- newtio.c_cc[VTIME] = 1;
- newtio.c_cc[VMIN] = 255;
- /*处理未接收字符*/
- tcflush(fd, TCIFLUSH);
- /*激活新配置*/
- if((tcsetattr(fd, TCSANOW, &newtio))!=0)
- {
- perror("com set error!");
- return -1;
- }
- printf("Set done!\n");
- return 0;
- }
- int open_port(int comport)
- {
- int fd = -1;
- switch(comport)
- {
- case 0:
- {
- fd = open("/dev/ttyO0", O_RDWR|O_NOCTTY|O_NDELAY);
- if(-1 == fd)
- {
- perror("Can't open Serial Port");
- return -1;
- }
- }
- break;
- case 1:
- {
- fd = open("/dev/ttyO1", O_RDWR|O_NOCTTY|O_NDELAY);
- if(-1 == fd)
- {
- perror("Can't open Serial Port");
- return -1;
- }
- }
- break;
- case 2:
- {
- fd = open("/dev/ttyO2", O_RDWR|O_NOCTTY|O_NDELAY);
- if(-1 == fd)
- {
- perror("Can't open Serial Port");
- return -1;
- }
- }
- break;
- case 3:
- {
- fd = open("/dev/ttyO3", O_RDWR|O_NOCTTY|O_NDELAY);
- if(-1 == fd)
- {
- perror("Can't open Serial Port");
- return -1;
- }
- }
- break;
- }
- /*恢复串口为阻塞状态*/
- if(fcntl(fd, F_SETFL, 0)<0)
- printf("fcntl failed!\n");
- else
- printf("fcntl=%d\n", fcntl(fd,F_SETFL,0));
- /*测试是否为终端设备*/
- if(isatty(STDIN_FILENO) == 0)
- printf("standard input is not a terminal device \n");
- else
- printf("isatty success!\n");
- printf("fd-open=%d\n",fd);
- return fd;
- }
- int main(int argc,char *argv[])
- {
- int fd,fdcom;
- int nread,maxd,nwrite,m,zread,zwrite,tmp,temp,i,j,fs_sel;
- fd_set fs_read;
- char buff[20],buffer[20];// = "comchecks";
- fdcom = open_port(atoi(argv[1]));
- if(fdcom<0)
- {
- perror("Open_prot 2 error");
- return;
- }
- //printf("com handle: %d \n", fdcom);
- if(argv[1]!=NULL)
- {
- fd = open_port(atoi(argv[1]));
- if(fd<0)
- {
- perror("Open_prot error");
- return;
- }
- }
- if(argv[2]!=NULL)
- {
- temp=set_opt(fdcom,atoi(argv[2]), 8, 'N', 1);
- if(temp<0)
- {
- perror("Set_opt ttyO2 error!");
- return;
- }
- tmp=set_opt(fd,atoi(argv[2]), 8, 'N', 1);
- if(tmp<0)
- {
- perror("Set_opt error!");
- return;
- }
- }
- else
- {
- temp=set_opt(fdcom,115200,8,'N', 1);
- if(temp<0)
- {
- perror("Set_opt ttyO2 error!");
- return;
- }
- tmp=set_opt(fd,115200,8,'N', 1);
- if(tmp<0)
- {
- perror("Set_opt error!");
- return;
- }
- }
- for(j=0;j<20;)
- {
- nwrite = write(fdcom,"fluarttest",10);//buff,strlen(buff));
- printf("j=%d, send is %d fluarttest\n",j,nwrite);
- lseek(fdcom,0,SEEK_SET);
- zread=read(fdcom,buffer,10);
- if(zread<0)
- {
- printf("zread error!\n");
- }
- else
- {
- char tmp[64];
- sprintf(tmp,"0x%x",(unsigned int)(buffer[0]));
- printf("tmp %s\n",tmp);
- }
-
- }
- close(fd);
- close(fdcom);
- return;
- }
复制代码 |
|