- 积分
- 27
贡献41
飞刀6 FD
注册时间2019-11-4
在线时间21 小时
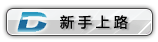
扫一扫,手机访问本帖 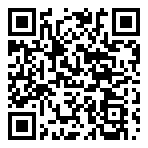
|
以下代码
- #include <unistd.h>
- #include <stdio.h>
- #include <stdlib.h>
- #include <fcntl.h>
- #include <string.h>
- #include <linux/fb.h>
- #include <sys/mman.h>
- #include <sys/ioctl.h>
- #include <arpa/inet.h>
- int main ( int argc, char *argv[] )
- {
- int fbfd = 0;
- struct fb_var_screeninfo vinfo;
- struct fb_fix_screeninfo finfo;
- long int screensize = 0;
- struct fb_bitfield red;
- struct fb_bitfield green;
- struct fb_bitfield blue;
-
- //打开显示设备
- fbfd = open("/dev/fb0", O_RDWR);
- if (!fbfd)
- {
- printf("Error: cannot open framebuffer device.\n");
- exit(1);
- }
-
- if (ioctl(fbfd, FBIOGET_FSCREENINFO, &finfo))
- {
- printf("Error:reading fixed information.\n");
- exit(2);
- }
-
- if (ioctl(fbfd, FBIOGET_VSCREENINFO, &vinfo))
- {
- printf("Error: reading variable information.\n");
- exit(3);
- }
-
- printf("R:%d,G:%d,B:%d \n", vinfo.red, vinfo.green, vinfo.blue );
-
- printf("%dx%d, %dbpp\n", vinfo.xres, vinfo.yres, vinfo.bits_per_pixel );
- xres = vinfo.xres;
- yres = vinfo.yres;
- bits_per_pixel = vinfo.bits_per_pixel;
-
- //计算屏幕的总大小(字节)
- screensize = vinfo.xres * vinfo.yres * vinfo.bits_per_pixel / 8;
- printf("screensize=%d byte\n",screensize);
-
- //对象映射
- fbp = (char *)mmap(0, screensize, PROT_READ | PROT_WRITE, MAP_SHARED, fbfd, 0);
- if ((int)fbp == -1)
- {
- printf("Error: failed to map framebuffer device to memory.\n");
- exit(4);
- }
-
- printf("sizeof file header=%d\n", sizeof(BITMAPFILEHEADER));
-
- printf("into show_bmp function\n");
-
- //显示图像
- show_bmp();
-
- //删除对象映射
- munmap(fbp, screensize);
- close(fbfd);
- return 0;
- }
|
|